DISPLAY DATA IN THE GRIDVIEW
The following code will help to display the data in the grid view.
The following code will help to display the data in the grid view.
private void getData()
{
//Here TestEntities is the class which is given from "Save entity connection setting in web.config"
TestEntities context = new TestEntities();
var query = from data in context.Employee
orderby data.name
select data;
//Bind Data to Gridview
grdEmployeeData.DataSource = query;
grdEmployeeData.DataBind();
}
Output
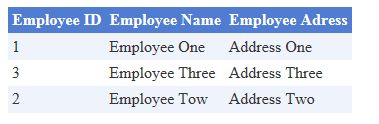
INSERT DATA IN THE EMPLOYEE TABLE
Use the following code to add the new employee:
private void insertData()
{
//Here TestEntities is the class which is given from "Save entity connection setting in web.config"
TestEntities context = new TestEntities();
// Create a new employee
Employee objEmployee = new Employee();
objEmployee.name = "Employee Four";
objEmployee.address = "Address Four";
//Add the created Employee object to context.
context.AddToEmployee(objEmployee);
context.SaveChanges();
}
Output
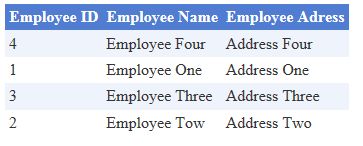
UPDATE DATA IN THE DATABASE
You can use the following code to update the employee details:
public void updateData()
{
//Here TestEntities is the class which is given from "Save entity connection setting in web.config"
TestEntities context = new TestEntities();
var query = from data in context.Employee
orderby data.name
select data;
foreach (Employee details in query)
{
if (details.id == 1)
{
//Assign the new values to name whose id is 1
details.name = "Updated Employee One";
}
}
//Save the changes back to database.
context.SaveChanges();
}
Output
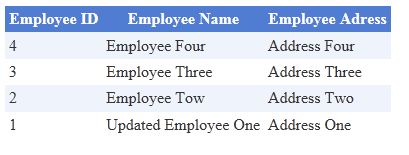
DELETE THE EMPLOYEE DETAILS FROM DATABASE
You can use the following code to delete the employee details from the database:
public void deleteData()
{
//Here TestEntities is the class which is given from "Save entity connection setting in web.config"
TestEntities context = new TestEntities();
var query = (from data in context.Employee
where data.id == 1
orderby data.name
select data).First();
context.Attach(query);
//DeleteObject is used to the delete the entity onject.
context.DeleteObject(query);
context.SaveChanges();
}
OUTPUT
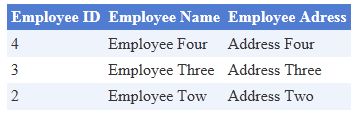
OUTPUT
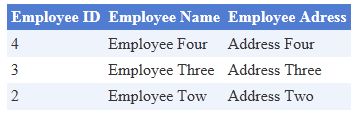